Graph API Sharepoint Operations
Microsoft provides Grap API to interact and work with Office 365 and Microsoft Cloud services. It allows to upload files, create and manage folders and use other services programmaticaly. In this article, I will show you how to perform basic operations on it using C#.
To start, you need to download nuget packages – Microsoft.Graph, Azure.Identity. They will allow us to make easy sharepoint calls and provide us all necessary Sharepoint models.
To create a connection beetween sharepoint and application you need to provide some informations. At first you need to build a token. First step is to create an IdentityClient.
Creating connection with sharepoint :
public async Task<AuthenticationToken?> GetAuthenticationToken()
{
var _identityClient = PublicClientApplicationBuilder
.Create("ENTER-APPLICATION-GUID")
.WithAuthority(AzureCloudInstance.AzurePublic, "common")
.WithRedirectUri("ENTER-REDIRECT-URL")
.Build();
var accounts = await _identityClient.GetAccountsAsync();
AuthenticationResult? result = null;
bool tryInteractiveLogin = false;
try
{
result = await _identityClient
.AcquireTokenSilent([ "User.Read", "Sites.Read.All", "Sites.ReadWrite.All" ], accounts.FirstOrDefault())
.ExecuteAsync();
}
catch (MsalUiRequiredException)
{
tryInteractiveLogin = true;
}
catch (Exception ex)
{
var message = ex.Message;
logger?.LogError("MSAL Silent Error: {message}", message);
}
if (tryInteractiveLogin)
{
try
{
result = await _identityClient
.AcquireTokenInteractive(_settings?.Scopes)
.ExecuteAsync();
}
catch (Exception ex)
{
var message = ex.Message;
logger?.LogError("MSAL Interactive Error: {message}", message);
}
}
if (result?.AccessToken != null)
{
_token = BuildToken(result);
}
else
{
logger?.LogInformation("MSAL Authentication Failed. Missing access token");
}
return _token;
}
At the begining, you need to create a PublicClientApplicationBuilder class instance. To get application Id, you need to follow below steps:
1. Go to portal.azure.com,
2. Search for “Microsoft Entra ID”,
3. From menu choose Enterprise applications or App Registrations.
4. Choose application,
5. Copy Application ID and paste it in code.
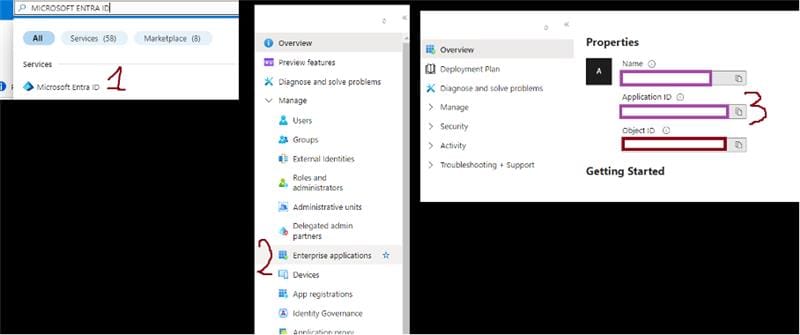
Then you retrieve all available accounts in the user token cache in application. Then we attempt to acquire an access token for the account from the user token cache. The access token is considered a match, if it contains at least all the requested scopes. That mean that an access token with more scopes than requested could be returned as well If the access token is expired or close to expiration, then the cached refresh token (if available) is used to acquire a new access token by making a silent network call. We can use previously created token to initialize graphClient instance.
var token = await _authService.GetAuthenticationToken();
TokenProvider provider = new TokenProvider(token.Value.Token);
var authenticationProvider = new BaseBearerTokenAuthenticationProvider(provider);
_graphClient = new GraphServiceClient(authenticationProvider);
Basic sharepoint operations
Firstly we need to get drive. Drive is top-level object, that represents user’s document library or OneDrive in Sharepoint. To do that, we can use following code:
var drive = await _graphClient.Sites[{siteId}].Lists[{listId}].Drive.GetAsync();
To get list ID you need to:
· Open your SharePoint site in a web browser
· Click gear icon in top-right
· Select Site Information and View all site settings
· Sites libraries and lists in Site Administration section
· Choose document library you want to get list from
· Get id from URL

To get site ID you need to:
· Open sharepoint
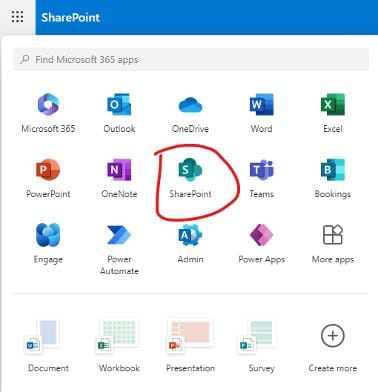
· Choose site
· Change URL, by adding /_api/site at the end

· Press ctrl + f, write d:id and copy value

· Create folder
To add new folder in root you need to retrieve it first. You can do it by using:
var root = await _graphClient.Drives[drive.Id].Root.GetAsync();
Later, we must initialize new DriveItem class object:
var requestBody = new DriveItem
{
Name = {folder-Name},
Folder = new Microsoft.Graph.Models.Folder
{
},
AdditionalData = new Dictionary<string, object>
{
{
"@microsoft.graph.conflictBehavior" , "fail"
},
},
};
Conflict Behaviours tells us, what to do when folder with the same name already exists. You can use fail, replace or rename. To create it in sharepoint, you can use following code:
await _graphClient.Drives[drive.Id].Items[root.Id].Children.PostAsync(requestBody);
You can pass another folder ID in Items, to create folder in it. After that operation, folder is created.
· Create File
To add file, you basically do same operation like in folder with small difference. While initializing DriveItem, instead of folder, you initialize File, like below:
var requestBody = new DriveItem
{
Name = file.FileName,
File = new FileObject
{
},
AdditionalData = new Dictionary<string, object>
{
{
"@microsoft.graph.conflictBehavior" , "replace"
},
},
};
var file = await _graphClient.Drives[drive.Id].Items[{folderId}].Children.PostAsync(requestBody);
To add content to it, you can update your file after creating it, using following code
await _graphClient.Drives[drive.Id].Items[file.Id].Content.PutAsync({content});
· Retrieve
To retrieve all items from folder, you can use following code:
var folders = await _graphClient.Drives[drive.Id]
.Items[{folder-id}]
.Children
.GetAsync()
You can also retrieve items using filter
var folders = await _graphClient.Drives[drive.Id]
.Items[{folder-id}]
.Children
.GetAsync(requestBuilder=>{
requestBuilder.QueryParameters.Filter = $"Name eq '{name}'"; })
More about query parameters here:
https://learn.microsoft.com/en-us/graph/query-parameters?tabs=csharp